What is SpringAI? GenAI App Dev Made Easy for Java Developers
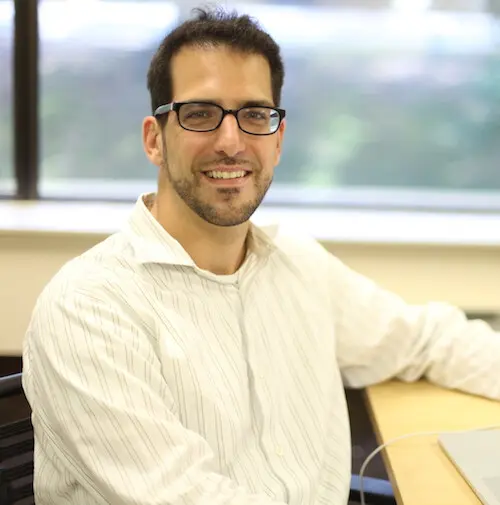
Russ Danner
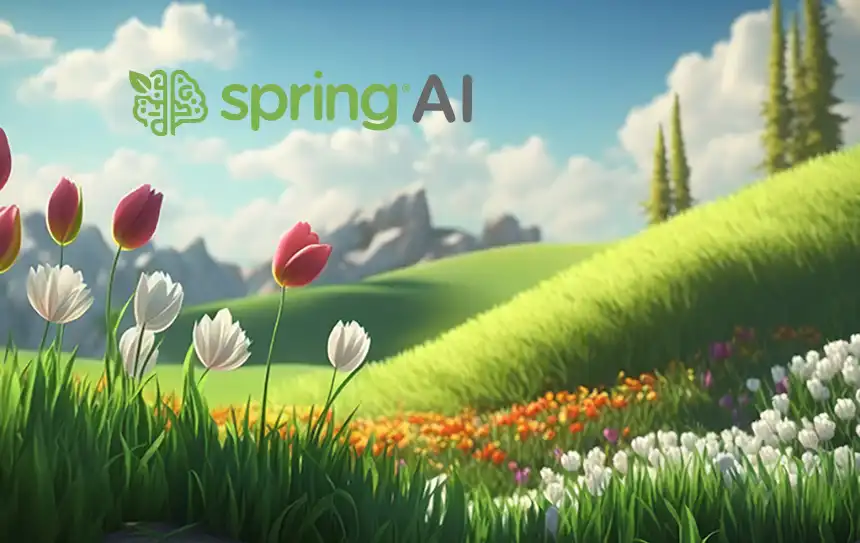
As Large Language Models (LLMs) and Generative AI (GenAI) reshape application development, Java developers face a growing need to integrate AI functionality—such as prompt engineering, chat-driven interfaces, and text generation—into their Spring-based applications. SpringAI is a new project from the Spring ecosystem that dramatically simplifies the integration of generative AI services with Spring Boot, allowing developers to leverage LLMs from providers like OpenAI, Azure OpenAI, Hugging Face, Amazon Bedrock, among others, using familiar Spring conventions.
In this blog post, we’ll explore the key features and benefits of SpringAI, why it matters to Java developers, and how you can get started building AI-driven applications with minimal friction.
Overview: What is SpringAI?
At its core, SpringAI is a Spring Boot integration framework that abstracts away the complexities of calling generative AI services. It includes:
- Clients and Connectors for various AI services (OpenAI, Hugging Face, Azure OpenAI, etc.).
- Prompt Handling and Templates to manage prompts in a structured, repeatable, and maintainable fashion.
- Chain-of-Responsibility style features (similar to “LangChain”) that allow you to sequence AI calls or combine them with external services.
- Observability hooks to monitor and optimize your AI calls.
For Java developers who are accustomed to the “Spring way” of building applications—through annotation-driven configurations, dependency injection, and autoconfiguration—SpringAI fits seamlessly into the existing development ecosystem.
Why Should Java Developers Care?
Familiar Spring Patterns
SpringAI’s biggest draw is that it feels like Spring. You can configure AI-related services the same way you would configure a data source, an external API client, or a message queue. This consistency means:
- Reduced Learning Curve: You don’t have to learn a brand-new paradigm; standard Spring Boot patterns (e.g., @Configuration, @Bean, application.properties/application.yml settings) apply.
- Boot Autoconfiguration: SpringAI autoconfigures itself if it detects your AI credentials or endpoints, streamlining setup.
- Dependency Injection: AI clients can be injected into your services and controllers using @Autowired, simplifying usage across your application.
Multiple AI Providers
SpringAI supports various providers right out of the box:
- OpenAI (e.g., GPT-4)
- Azure OpenAI (a managed offering of OpenAI’s models on Microsoft Azure)
- Hugging Face (community models and endpoints)
- Amazon Bedrock (including Cohere and Titan models)
- Stability AI (for text to image generation)
- and many more.
This flexibility lets you switch AI vendors or experiment with multiple providers without rewriting large portions of your code.
Prompt Management and Templating
Generative AI is only as good as the prompts you feed it. SpringAI offers:
- Prompt Templates: Make your prompts more dynamic and version-controlled. You can embed placeholders for variables (e.g., user input, query context) and avoid repetitive string concatenation.
- Reusability: You can create a library of prompt templates that can be injected anywhere in your application.
- Ease of Maintenance: If a prompt needs updating, you can modify a single template rather than hunting through multiple code paths.
Chain of Tasks
Modern LLM usage often involves chaining different AI tasks (like summarizing, then translating, then formatting results) or combining AI calls with external services (search, databases, APIs). SpringAI helps orchestrate these steps in a composable manner:
- Chain-of-Responsibility Pattern: Organize sequences of AI calls.
- Spring Boot Integration: Each step in the chain can be tied to a Spring-managed bean or service, simplifying dependency management.
- Mixed Execution: Insert traditional business logic (e.g., query a database) between AI calls in a single pipeline.
Reactive and Streaming Support
For applications using Spring WebFlux, SpringAI supports:
- Reactive Programming: Asynchronous, non-blocking AI calls that can handle large throughput.
- Streaming: If the AI provider supports server-sent events (SSE), you can stream partial completions in real-time, enabling chat-like experiences or live content generation.
Security and Observability
SpringAI respects the standard Spring Boot security, encryption, and logging best practices. You can:
- Store and Encrypt API Keys securely in properties or environment variables.
- Leverage Spring’s Observability Stack (Micrometer, etc.) to track metrics like API call frequency, response latency, and token usage.
- Centralize Configuration: Manage AI provider credentials using the same method you use for other sensitive configs, like database passwords or API keys.
Integration with Existing Spring Projects
Lastly, SpringAI is designed to fit into your existing microservices or monoliths with minimal disruption:
- Spring Data: Combine AI-driven logic with your database queries and repositories.
- Spring Cloud: Deploy to a cloud environment (e.g., Kubernetes) with scaling policies that handle unpredictable spikes from AI-driven workloads.
- Spring Security: Restrict usage of AI endpoints or functionalities to authorized users, ensuring compliance and controlling costs.
- Crafter Engine: Add generative AI functionality to your CrafterCMS-powered sites and apps by building on this Spring-based, headless content delivery platform.
Key Components of SpringAI
- AI Client Libraries: These handle the low-level API calls and authentication to providers like OpenAI or Azure OpenAI. Developers can configure them via application.yml or environment variables.
- LLM Services: Abstractions like CompletionService or ChatService let you request text completions, chat interactions, or function calling without manually crafting HTTP requests or handling tokens.
- Prompt Templates and Builders
- The Template or builder approach allows you to define placeholders and pass dynamic content at runtime.
- This ensures cleanliness and maintainability of complex, multi-line prompts.
- Chaining and Pipelines
- A mechanism for linking multiple AI calls (or AI calls + external services) in a single flow.
- Facilitates advanced scenarios like retrieval-augmented generation (RAG) by injecting external context between AI calls.
- Integration Points
- Annotations for injecting LLM clients and defining chain steps.
- Properties for easy dev/test/prod environment setup.
Quick Example: Setting up SpringAI
Below is a hypothetical snippet to give you a taste of how SpringAI might fit into a Spring Boot app:
// application.yml
spring:
ai:
openai:
api-key: ${OPENAI_API_KEY}
base-url: https://api.openai.com/v1
// A simple service using SpringAI
@Service
public class MyAiService {
private final CompletionService completionService;
// SpringAI auto-configuration injects the appropriate LLM client
public MyAiService(CompletionService completionService) {
this.completionService = completionService;
}
public String generateSummary(String text) {
// Create a prompt template
String prompt = "Summarize the following text in one paragraph:\n" + text;
// Call the LLM
CompletionRequest request = CompletionRequest.builder()
.prompt(prompt)
.maxTokens(150)
.build();
return completionService.complete(request).getValue();
}
}
With a few lines of YAML and some basic code, you’re ready to integrate AI-powered text generation. All of the complexities—authentication, error handling, and streaming—are handled under the hood by SpringAI.
Getting Started with SpringAI
To experiment with SpringAI in your own project:
- Add the SpringAI Dependency
<dependency> <groupId>org.springframework.ai</groupId> <artifactId>spring-ai</artifactId> <version>YOUR_VERSION_HERE</version> </dependency>
- Configure the Provider
In application.yml or application.properties, provide your OpenAI, Azure, or Hugging Face credentials and base URLs. - Inject and Use
Inject the AI service beans in your Controllers/Services to start making requests. - Iterate and Optimize
-
- Fine-tune your prompts.
- Experiment with chaining logic.
- Monitor usage and cost.
Conclusion
SpringAI brings the power of generative AI into the familiar realm of Spring Boot, letting Java developers harness LLMs without having to master complex APIs or drastically alter their existing development workflows. Whether you need to generate text, build interactive chatbots, or orchestrate multi-step AI pipelines, SpringAI provides a clean, Spring-like approach to AI integration.
By lowering the barrier to entry for AI features, SpringAI empowers Java teams to iterate faster, innovate on new AI-driven use cases, and remain focused on core business logic instead of wrestling with low-level AI service details. For any Spring-based project looking to add generative AI capabilities—whether for content creation, code suggestions, or advanced user interactions—SpringAI is a compelling new toolkit that should be on your radar.
Next Steps
- Check out the SpringAI Reference Docs for detailed setup instructions, examples, and best practices.
- Experiment with a Hello LLM project locally to get a feel for prompt templates and completions.
- Explore advanced chaining scenarios and integrate with external data sources to build full-fledged AI-driven applications.
- Download CrafterCMS along with SpringAI and start adding generative AI functionality – such as chatbots, product discovery guides, and customer onboarding and support helpdesk – to your next-generation websites, e-commerce experiences, and other digital experience apps.
Happy AI coding, and welcome to the next frontier of Java/Spring development!